Deploying WordPress and MySQL on Kubernetes using Terraform
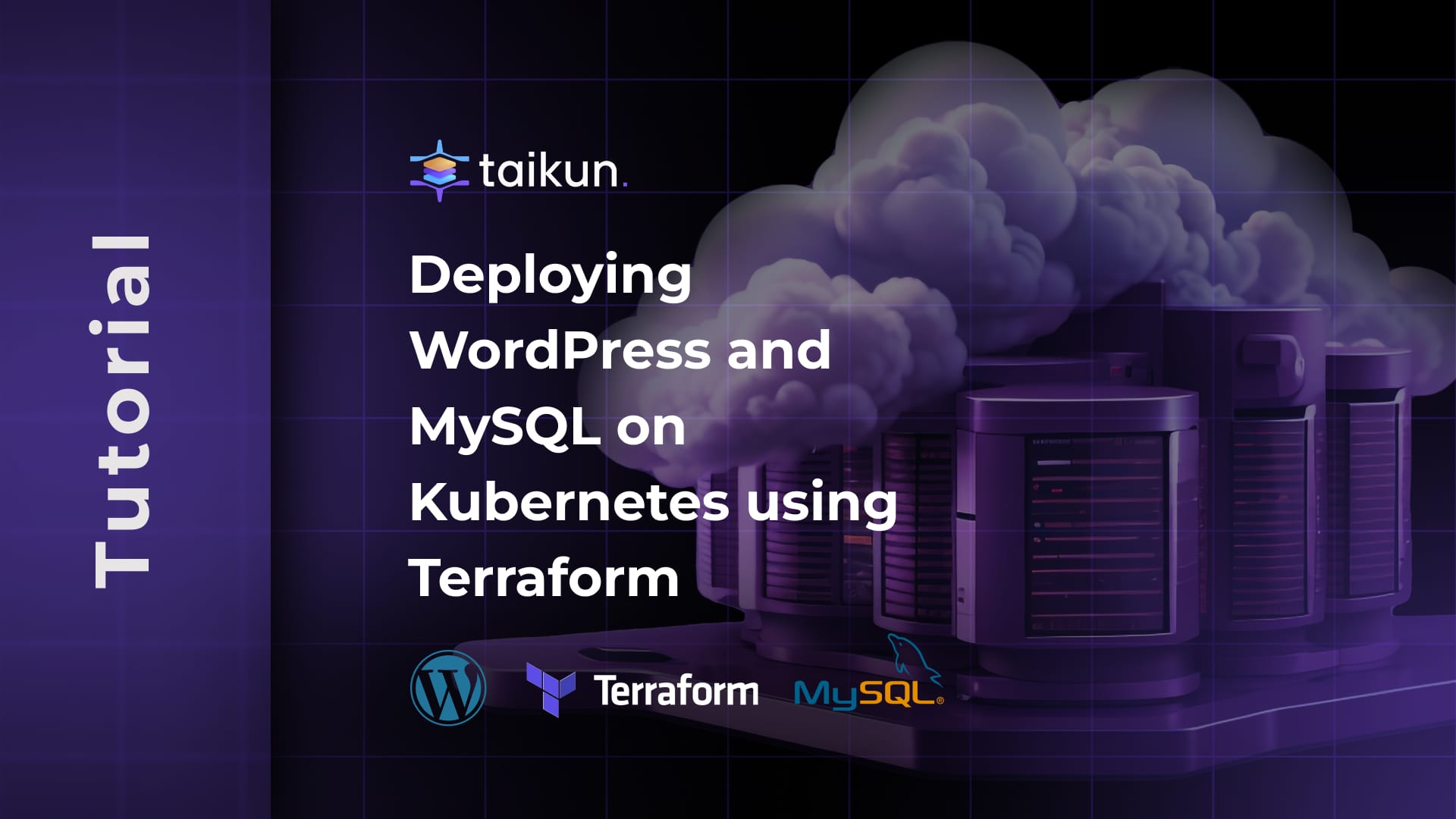
In this guide, we'll walk through deploying a WordPress application and MySQL for Database on Taikun CloudWorks using Terraform. This approach enables infrastructure as code and allows for consistent, repeatable deployments with just a single click.
Prerequisites
- Terraform installed (version 0.12 or later)
- Taikun CloudWorks account
- Basic understanding of Terraform and Kubernetes
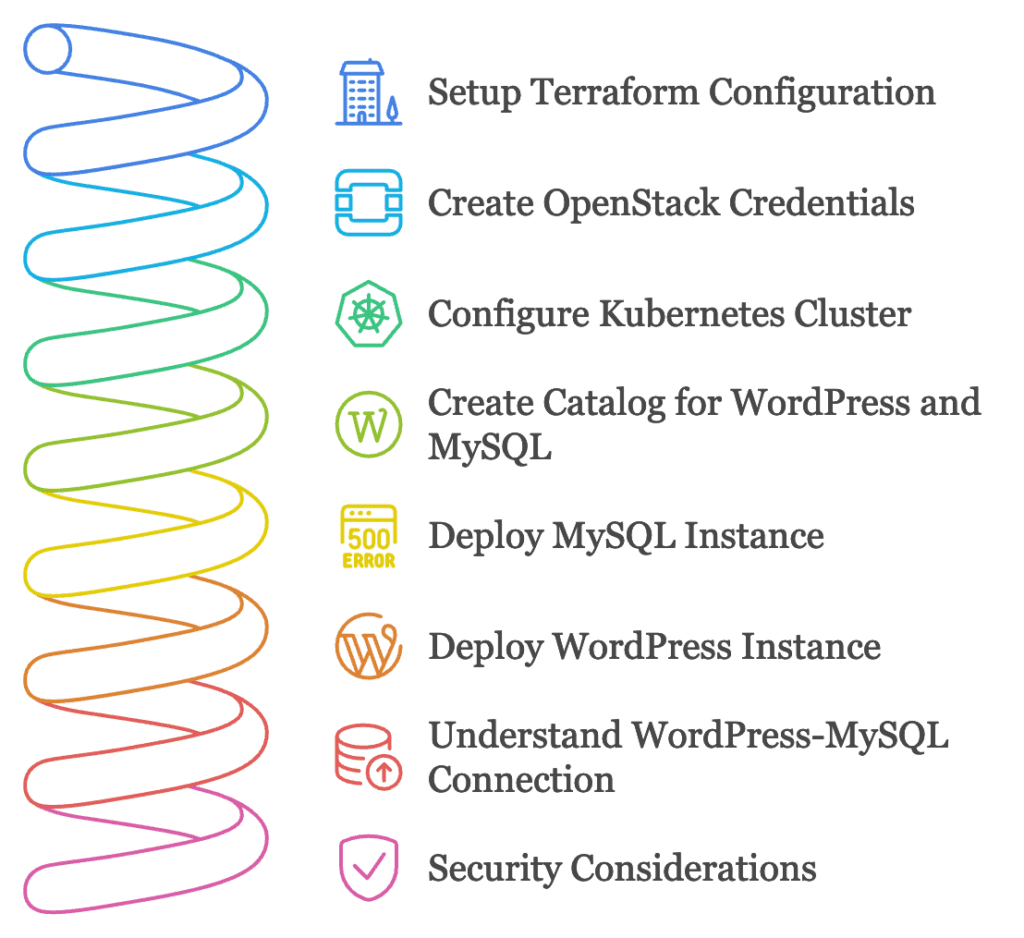
Setting Up the Terraform Configuration
First, let's set up our Terraform configuration with the required provider:
terraform {
required_providers {
taikun = {
source = "itera-io/taikun"
version = "1.9.1" // Use the latest version
}
}
}
// Define connection to Taikun CloudWorks
provider "taikun" {
email = "example@taikun.cloud"
password = "your-password"
}
Creating OpenStack Cloud Credentials
We'll start by defining our OpenStack cloud credentials in Taikun CloudWorks:
resource "taikun_cloud_credential_openstack" "wordpress_credentials" {
name = "wordpress-openstack-cc"
user = "your-username"
password = "your-password"
url = "openstack-url"
domain = "your-domain"
project_name = "your-project"
public_network_name = "public-network"
region = "your-region"
lock = false
}
Configuring Kubernetes Cluster
Next, we'll create a Kubernetes cluster with the necessary components:
// Create a kubernetes cluster
resource "taikun_project" "proj1" {
name = "tf-acc-oneclick"
cloud_credential_id = taikun_cloud_credential_openstack.foo.id
flavors = concat(local.flavors_small, local.flavors_big)
server_bastion {
name = "tf-acc-bastion"
disk_size = 30
flavor = local.flavors_small[0]
}
server_kubemaster {
name = "tf-acc-master"
disk_size = 30
flavor = local.flavors_small[0]
}
server_kubeworker {
name = "tf-acc-worker"
disk_size = 100
flavor = local.flavors_big[0]
}
}
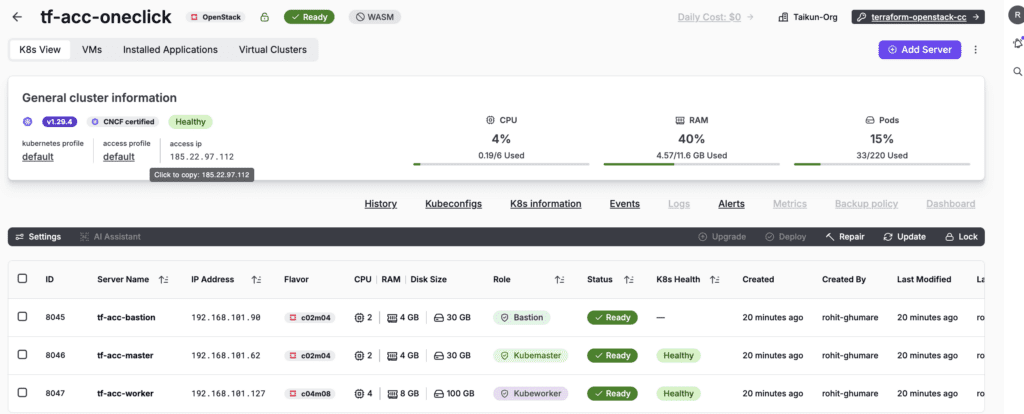
Creating a Catalog and Adding WordPress with MySQL
Now, let's create a catalog and add both WordPress and MySQL applications:
// Create a catalog with WordPress and MySQL applications
resource "taikun_catalog" "cat01" {
name = "oneclick-catalog"
description = "Created by terraform for oneclick deployment."
projects = [taikun_project.proj1.id]
application {
name = "wordpress"
repository = "taikun-managed-apps"
}
application {
name = "mysql"
repository = "taikun-managed-apps"
}
}
Deploying MySQL Instance
First, let's deploy the MySQL instance that WordPress will use:
// Create MySQL instance first
resource "taikun_app_instance" "mysql01" {
name = "terraform-mysql01"
namespace = "wordpress-ns"
project_id = taikun_project.proj1.id
catalog_app_id = local.mysql_app_id
depends_on = [taikun_project.proj1]
}
Deploying WordPress Instance with MySQL Connection
Now, let's deploy WordPress and configure it to use the MySQL instance:
// Create WordPress instance with MySQL connection
resource "taikun_app_instance" "wordpress01" {
name = "terraform-wp01"
namespace = "wordpress-ns"
project_id = taikun_project.proj1.id
catalog_app_id = local.wp_app_id
depends_on = [taikun_project.proj1, taikun_app_instance.mysql01]
}
// App ID selectors
locals {
wp_app_id = [for app in tolist(taikun_catalog.cat01.application) :
app.id if app.name == "wordpress" && app.repository == "taikun-managed-apps"][0]
mysql_app_id = [for app in tolist(taikun_catalog.cat01.application) :
app.id if app.name == "mysql" && app.repository == "taikun-managed-apps"][0]
}
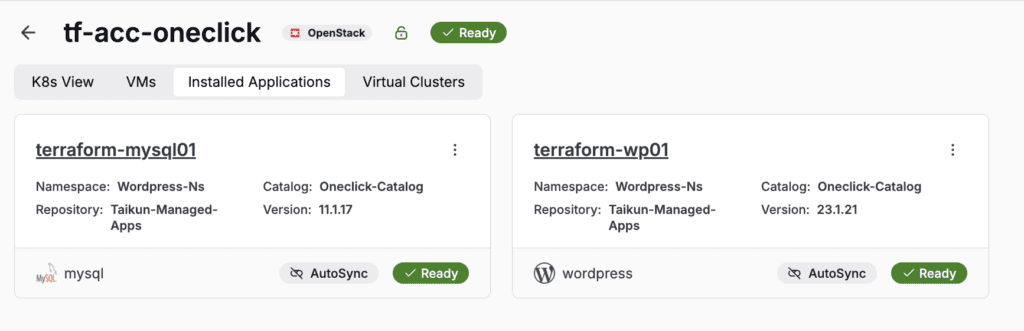
Understanding the WordPress-MySQL Connection
The configuration above sets up:
- A dedicated MySQL instance with:
- A root password
- A specific database for WordPress
- A dedicated user for WordPress
- Persistent storage for database files
- WordPress configured to:
- Use the external MySQL database instead of the built-in MariaDB
- Connect to MySQL using Kubernetes service DNS
- Use persistent storage for WordPress files
Connection Details
The MySQL connection is established using Kubernetes internal DNS. The format ${service_name}.${namespace}.svc.cluster.local
ensures WordPress can reliably connect to MySQL within the cluster.
Security Considerations
For production deployments, consider:
- Secrets Management:
- Use Kubernetes secrets or HashiCorp Vault for sensitive values
- Rotate database passwords regularly
- Use environment variables for sensitive configuration
- Database Security:
- Enable MySQL SSL/TLS connections
- Implement database backup strategies
- Set up proper database user permissions
- Network Security:
- Use network policies to restrict pod-to-pod communication
- Enable MySQL bind-address restrictions
- Implement proper firewall rules
Flavor Configuration
Define the compute resources for your cluster:
// Flavors configuration. Filtering data sources
data "taikun_flavors" "small" {
cloud_credential_id = taikun_cloud_credential_openstack.foo.id
min_cpu = 2
max_cpu = 2
min_ram = 4
max_ram = 4
}
data "taikun_flavors" "big" {
cloud_credential_id = taikun_cloud_credential_openstack.foo.id
min_cpu = 4
max_cpu = 4
min_ram = 8
max_ram = 8
}
locals {
flavors_small = [for flavor in data.taikun_flavors.small.flavors : flavor.name]
flavors_big = [for flavor in data.taikun_flavors.big.flavors : flavor.name]
}
Deployment Steps
- Save all the above configurations in a
.tf
file - Initialize Terraform:
terraform init
- Review the deployment plan:
terraform plan
- Apply the configuration:
terraform apply
Expected Output:
Do you want to perform these actions?
Terraform will perform the actions described above.
Only 'yes' will be accepted to approve.
Enter a value: yes
taikun_cloud_credential_openstack.foo: Creating...
taikun_cloud_credential_openstack.foo: Creation complete after 3s [id=1240]
data.taikun_flavors.small: Reading...
data.taikun_flavors.big: Reading...
data.taikun_flavors.big: Read complete after 1s [id=1240]
data.taikun_flavors.small: Read complete after 1s [id=1240]
taikun_project.proj1: Creating...
taikun_project.proj1: Still creating... [10s elapsed]
taikun_project.proj1: Still creating... [20s elapsed]
taikun_project.proj1: Still creating... [30s elapsed]
taikun_project.proj1: Still creating... [40s elapsed]
taikun_project.proj1: Still creating... [50s elapsed]
taikun_project.proj1: Still creating... [1m0s elapsed]
.
.
.
taikun_project.proj1: Still creating... [9m0s elapsed]
taikun_project.proj1: Creation complete after 9m8s [id=2322]
taikun_catalog.cat01: Creating...
taikun_catalog.cat01: Creation complete after 4s [id=652]
taikun_app_instance.mysql01: Creating...
taikun_app_instance.mysql01: Still creating... [10s elapsed]
taikun_app_instance.mysql01: Still creating... [20s elapsed]
taikun_app_instance.mysql01: Still creating... [30s elapsed]
taikun_app_instance.mysql01: Still creating... [40s elapsed]
taikun_app_instance.mysql01: Still creating... [50s elapsed]
taikun_app_instance.mysql01: Still creating... [1m0s elapsed]
taikun_app_instance.mysql01: Still creating... [1m10s elapsed]
taikun_app_instance.mysql01: Still creating... [1m20s elapsed]
taikun_app_instance.mysql01: Still creating... [1m30s elapsed]
taikun_app_instance.mysql01: Creation complete after 1m37s [id=1653]
taikun_app_instance.wordpress01: Creating...
taikun_app_instance.wordpress01: Still creating... [10s elapsed]
taikun_app_instance.wordpress01: Still creating... [20s elapsed]
taikun_app_instance.wordpress01: Still creating... [30s elapsed]
taikun_app_instance.wordpress01: Still creating... [40s elapsed]
taikun_app_instance.wordpress01: Still creating... [50s elapsed]
taikun_app_instance.wordpress01: Still creating... [1m0s elapsed]
taikun_app_instance.wordpress01: Still creating... [1m10s elapsed]
taikun_app_instance.wordpress01: Still creating... [1m20s elapsed]
taikun_app_instance.wordpress01: Still creating... [1m30s elapsed]
taikun_app_instance.wordpress01: Still creating... [1m40s elapsed]
taikun_app_instance.wordpress01: Creation complete after 1m47s [id=1654]
Apply complete! Resources: 5 added, 0 changed, 0 destroyed.
What Happens Behind the Scenes?
When you run terraform apply
, the following actions occur:
- Adds OpenStack cloud credentials in Taikun CloudWorks
- Provisions a Kubernetes cluster with:
- A bastion host for secure access
- A Kubernetes master node
- A worker node for running applications
- Sets up a catalog with WordPress and MySQL applications
- Deploys WordPress with MySQL using Taikun Cloudworks's managed apps
Accessing Your WordPress Site
Once the deployment is complete, you can access your WordPress site through the URL provided by CloudWorks. The URL will be available in your CloudWorks dashboard or through the Terraform output.
WordPress URL can be accessed by executing commands found in the WordPress application logs, as illustrated below:
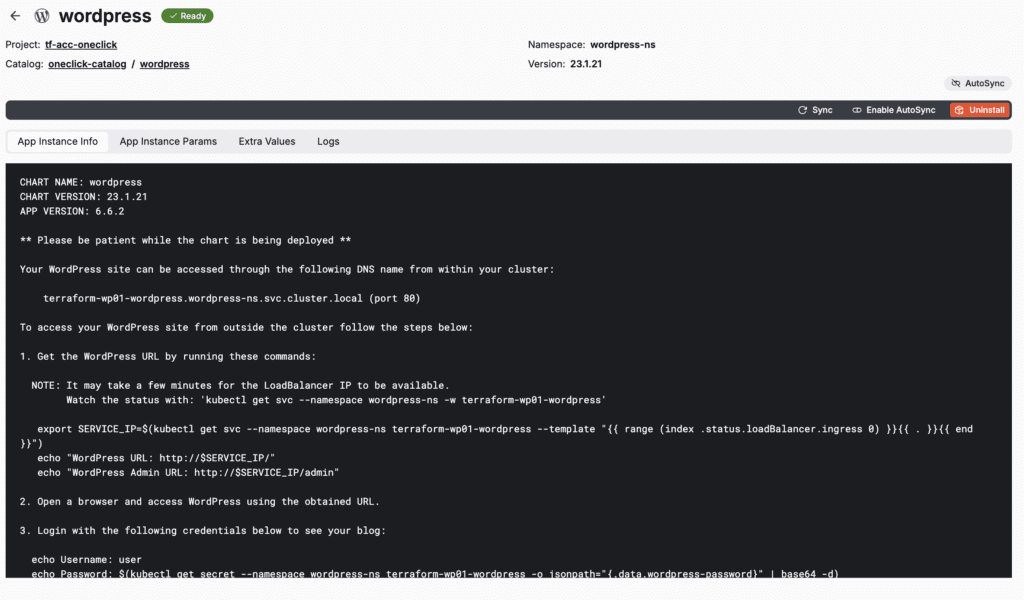
In Taikun CloudWorks, create a Kubeconfig to access the terminal, or download the Kubeconfig for later use.
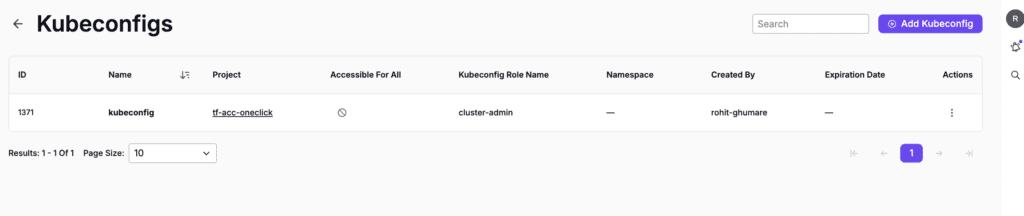
Access the URL
here
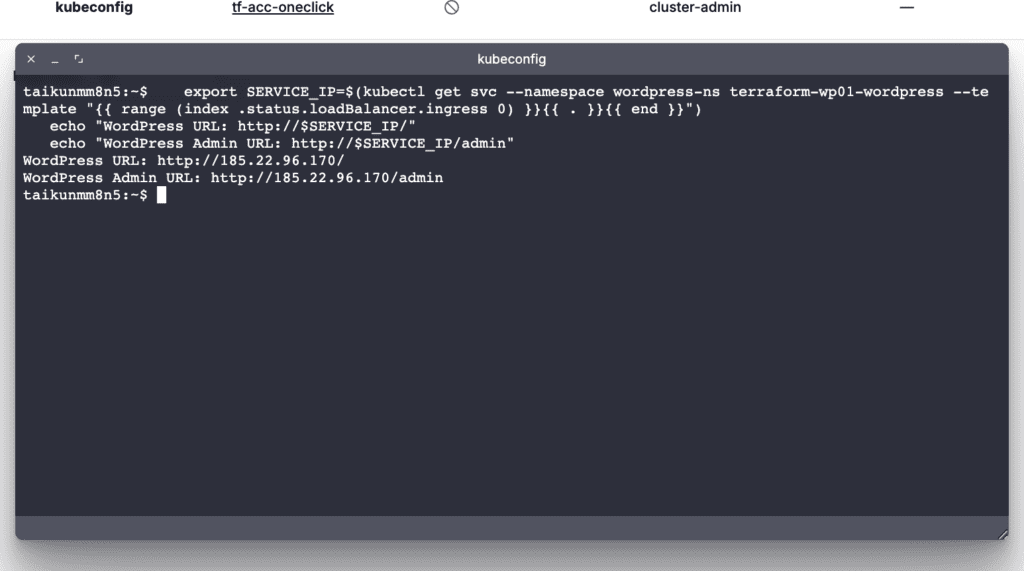
Output
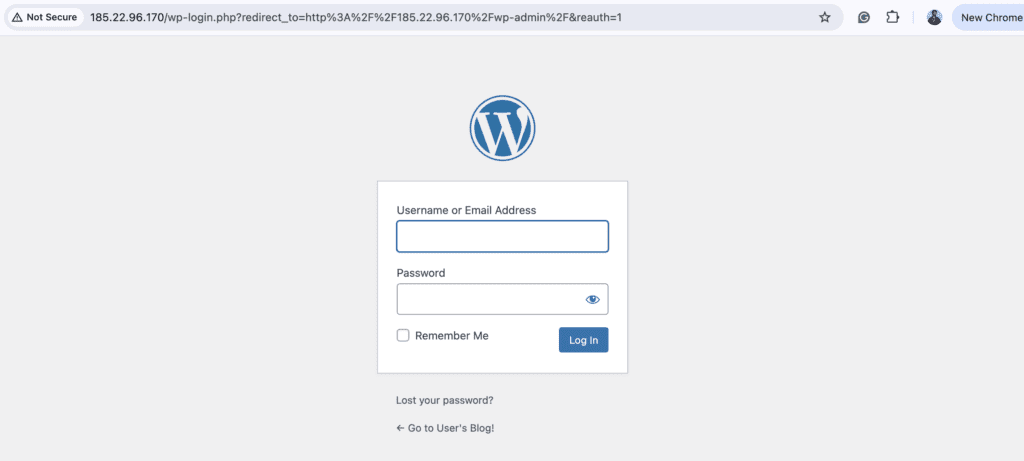
Clean Up
To remove all resources, run:
terraform destroy

The Terraform script for application setup on CloudWorks is available in our GitHub repository here: Sample Terraform script
Best Practices
- Security:
- Never commit credentials to version control
- Use environment variables or secure vaults for sensitive data
- Enable HTTPS for production deployments
- Resource Sizing:
- Adjust flavor sizes based on your workload requirements
- Consider using auto-scaling for production environments
- Backup:
- Implement regular backup solutions for your WordPress data
- Store backups in a separate location
Conclusion
Using Taikun Terraform Provider provides the easiest way to deploy WordPress applications. This infrastructure-as-code approach ensures consistency and repeatability while simplifying the deployment process to a single command.
Remember to consult the Taikun documentation for the latest updates and best practices for production deployments.
Taikun CloudWorks is a one-stop solution for your Kubernetes workloads. Try Taikun CloudWorks today. Book your free demo today, and let our team simplify, enhance, and streamline your infrastructure management.